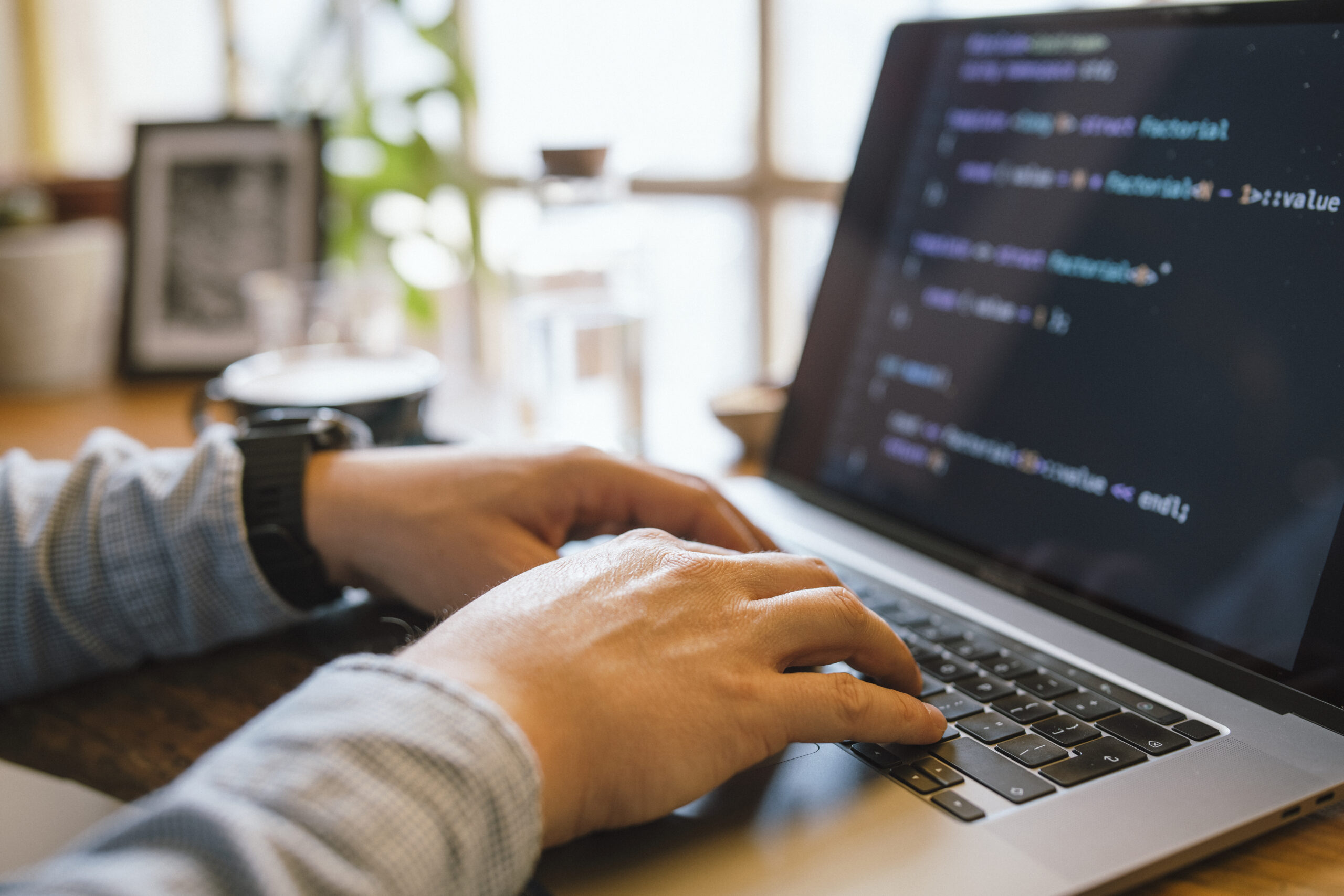
Debugging is One of the more important — nevertheless normally overlooked — abilities within a developer’s toolkit. It's actually not almost repairing damaged code; it’s about knowledge how and why factors go Mistaken, and Mastering to Imagine methodically to unravel complications competently. Regardless of whether you're a novice or even a seasoned developer, sharpening your debugging expertise can preserve hours of stress and radically help your efficiency. Here's various approaches that will help builders degree up their debugging recreation by me, Gustavo Woltmann.
Master Your Tools
On the list of quickest means builders can elevate their debugging expertise is by mastering the resources they use every day. While producing code is a person Component of growth, realizing how you can connect with it properly through execution is equally important. Fashionable growth environments come Geared up with impressive debugging capabilities — but numerous builders only scratch the surface area of what these applications can do.
Take, for instance, an Integrated Advancement Atmosphere (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These equipment permit you to set breakpoints, inspect the worth of variables at runtime, phase via code line by line, and perhaps modify code about the fly. When employed appropriately, they let you notice precisely how your code behaves all through execution, which can be invaluable for monitoring down elusive bugs.
Browser developer equipment, which include Chrome DevTools, are indispensable for front-close developers. They assist you to inspect the DOM, check network requests, look at real-time effectiveness metrics, and debug JavaScript within the browser. Mastering the console, resources, and community tabs can switch frustrating UI concerns into workable tasks.
For backend or program-amount developers, applications like GDB (GNU Debugger), Valgrind, or LLDB provide deep Management around operating processes and memory administration. Learning these instruments may have a steeper Understanding curve but pays off when debugging effectiveness issues, memory leaks, or segmentation faults.
Past your IDE or debugger, turn into comfortable with Edition Management devices like Git to understand code background, locate the precise instant bugs were introduced, and isolate problematic adjustments.
In the long run, mastering your instruments usually means likely past default options and shortcuts — it’s about building an intimate understanding of your growth natural environment so that when problems come up, you’re not misplaced at midnight. The better you understand your resources, the greater time it is possible to commit fixing the actual issue instead of fumbling via the method.
Reproduce the trouble
Just about the most essential — and sometimes ignored — actions in effective debugging is reproducing the trouble. Just before jumping into your code or building guesses, builders want to create a constant atmosphere or state of affairs wherever the bug reliably appears. With out reproducibility, correcting a bug will become a activity of probability, usually leading to squandered time and fragile code adjustments.
The first step in reproducing a dilemma is accumulating as much context as possible. Check with inquiries like: What actions brought about the issue? Which atmosphere was it in — enhancement, staging, or generation? Are there any logs, screenshots, or error messages? The greater detail you may have, the less difficult it turns into to isolate the precise problems under which the bug happens.
When you’ve gathered sufficient facts, endeavor to recreate the situation in your local natural environment. This could indicate inputting exactly the same facts, simulating related user interactions, or mimicking program states. If The difficulty appears intermittently, look at writing automated tests that replicate the edge conditions or condition transitions included. These tests not merely assistance expose the issue but will also avoid regressions Sooner or later.
Sometimes, The problem can be environment-certain — it'd occur only on specified functioning systems, browsers, or beneath unique configurations. Applying equipment like Digital equipment, containerization (e.g., Docker), or cross-browser tests platforms may be instrumental in replicating this sort of bugs.
Reproducing the situation isn’t simply a step — it’s a frame of mind. It involves tolerance, observation, and a methodical method. But after you can continually recreate the bug, you might be presently halfway to repairing it. Using a reproducible circumstance, You need to use your debugging instruments additional correctly, exam potential fixes safely, and communicate more Obviously with your group or buyers. It turns an abstract complaint into a concrete challenge — Which’s where builders prosper.
Examine and Have an understanding of the Mistake Messages
Mistake messages are often the most precious clues a developer has when anything goes Mistaken. In lieu of viewing them as aggravating interruptions, developers must understand to deal with error messages as direct communications in the procedure. They normally show you exactly what transpired, where by it took place, and often even why it transpired — if you know the way to interpret them.
Start off by reading through the message carefully As well as in entire. A lot of builders, specially when underneath time force, look at the primary line and right away begin producing assumptions. But deeper in the mistake stack or logs could lie the true root induce. Don’t just copy and paste error messages into search engines like google and yahoo — read and fully grasp them very first.
Crack the error down into pieces. Could it be a syntax mistake, a runtime exception, or even a logic error? Will it position to a specific file and line variety? What module or function activated it? These questions can tutorial your investigation and position you toward the dependable code.
It’s also useful to be familiar with the terminology in the programming language or framework you’re applying. Error messages in languages like Python, JavaScript, or Java normally stick to predictable patterns, and Mastering to recognize these can dramatically increase your debugging method.
Some glitches are vague or generic, and in those situations, it’s very important to examine the context through which the mistake occurred. Examine linked log entries, input values, and recent improvements in the codebase.
Don’t neglect compiler or linter warnings both. These generally precede larger sized problems and provide hints about opportunity bugs.
In the end, error messages will not be your enemies—they’re your guides. Mastering to interpret them the right way turns chaos into clarity, helping you pinpoint concerns more rapidly, reduce debugging time, and become a much more effective and assured developer.
Use Logging Properly
Logging is The most highly effective applications inside of a developer’s debugging toolkit. When utilised properly, it offers true-time insights into how an software behaves, supporting you recognize what’s occurring beneath the hood with no need to pause execution or move in the code line by line.
A very good logging system commences with figuring out what to log and at what stage. Widespread logging stages incorporate DEBUG, Details, Alert, ERROR, and FATAL. Use DEBUG for detailed diagnostic information and facts all through progress, Details for standard gatherings (like profitable start off-ups), WARN for potential challenges that don’t split the appliance, ERROR for precise complications, and Deadly once the method can’t go on.
Prevent flooding your logs with extreme or irrelevant information. Too much logging can obscure significant messages and decelerate your program. Deal with essential occasions, point out alterations, input/output values, and significant selection points in your code.
Structure your log messages clearly and continually. Contain context, which include timestamps, request IDs, and performance names, so it’s simpler to trace concerns in dispersed techniques or multi-threaded environments. Structured logging (e.g., JSON logs) will make it even simpler to parse and filter logs programmatically.
During debugging, logs Enable you to keep track of how variables evolve, what problems are achieved, and what branches of logic are executed—all with no halting the program. They’re In particular beneficial in generation environments exactly where stepping by way of code isn’t possible.
Moreover, use logging frameworks and applications (like Log4j, Winston, or Python’s logging module) that help log rotation, filtering, and integration with monitoring dashboards.
Eventually, clever logging is about balance and clarity. By using a perfectly-believed-out logging tactic, you can reduce the time it will require to spot troubles, attain deeper visibility into your programs, and Enhance the Over-all maintainability and reliability of one's code.
Consider Similar to a Detective
Debugging is not just a specialized undertaking—it is a form of investigation. To successfully discover and deal with bugs, builders must method the procedure similar to a detective resolving a secret. This mindset aids break down intricate difficulties into workable pieces and stick to clues logically to uncover the basis lead to.
Start off by collecting proof. Consider the signs or symptoms of the condition: mistake messages, incorrect output, or general performance concerns. Much like a detective surveys a criminal offense scene, gather as much appropriate data as it is possible to devoid of leaping to conclusions. Use logs, examination conditions, and person stories to piece jointly a transparent image of what’s happening.
Subsequent, form hypotheses. Ask you: What might be creating this behavior? Have any variations not long ago been designed on the codebase? Has this concern occurred right before underneath related situation? The purpose is always to narrow down alternatives and discover prospective culprits.
Then, test your theories systematically. Seek to recreate the situation within a controlled environment. When you suspect a particular function or component, isolate it and validate if The problem persists. Like a detective conducting interviews, ask your code issues and Allow the results guide you closer to the reality.
Shell out close awareness to tiny details. Bugs generally conceal during the the very least anticipated sites—just like a lacking semicolon, an off-by-a single mistake, or possibly a race condition. Be extensive and patient, resisting the urge to patch The problem without having absolutely knowing it. Non permanent fixes may possibly hide the true problem, just for it to resurface afterwards.
Finally, retain notes on That which you tried and uncovered. Equally as detectives log their investigations, documenting your debugging procedure can help save time for future concerns and enable others recognize your reasoning.
By wondering similar to a detective, developers can sharpen their analytical expertise, tactic problems methodically, and grow to be simpler at uncovering concealed issues in complicated programs.
Generate Exams
Crafting tests is one of the most effective approaches to transform your debugging competencies and overall improvement effectiveness. Exams not simply assistance catch bugs early but additionally serve as a safety Internet that provides you self confidence when generating improvements towards your codebase. A perfectly-tested software is easier to debug because it enables you to pinpoint specifically in which and when a difficulty happens.
Start with unit tests, which focus on individual functions or modules. These little, isolated tests can rapidly reveal whether a specific bit of logic is Performing as predicted. Each time a examination fails, you right away know exactly where to search, substantially decreasing the time used debugging. Device exams are Particularly useful for catching regression bugs—challenges that reappear immediately after Earlier getting mounted.
Subsequent, combine integration assessments and stop-to-finish checks into your workflow. These support make certain that various aspects of your application function alongside one another smoothly. They’re specially beneficial for catching bugs that occur in advanced techniques with numerous parts or providers interacting. If something breaks, your assessments can tell you which Component of the pipeline failed and below what disorders.
Composing checks also forces you to think critically regarding your code. To check a characteristic properly, you may need to know its inputs, predicted outputs, and edge instances. This volume of comprehension naturally qualified prospects to raised code construction and less bugs.
When debugging a difficulty, composing a failing exam that reproduces the bug may be a strong starting point. After the take a look at fails constantly, you may concentrate on repairing the bug and enjoy your test move when The problem is fixed. This method makes sure that the exact same bug doesn’t return in the future.
In brief, crafting tests turns debugging from a annoying guessing video game right into a structured and predictable procedure—aiding you capture additional bugs, faster and much more reliably.
Get Breaks
When debugging a difficult challenge, it’s simple to become immersed in the challenge—observing your monitor for several hours, trying Answer right after Remedy. But The most underrated debugging instruments is solely stepping absent. Getting breaks can help you reset your mind, decrease aggravation, and often see the issue from a new perspective.
If you're much too near the code for much too extensive, cognitive exhaustion sets in. You may perhaps get started overlooking noticeable errors or misreading code that you wrote just hours before. During this point out, your brain turns into significantly less effective at problem-resolving. A brief stroll, a coffee break, or perhaps switching to a different task for ten–15 minutes can refresh your target. Numerous builders report acquiring the basis of an issue after they've taken the perfect time to disconnect, allowing their subconscious function in the background.
Breaks also assistance protect against burnout, Specially throughout longer debugging classes. Sitting before a display screen, mentally stuck, is don't just unproductive but in addition draining. Stepping away helps you to return with renewed Strength along with a clearer state of mind. You may perhaps out of the blue discover a lacking semicolon, a logic flaw, or even a misplaced variable that eluded you before.
When you’re stuck, a fantastic rule of thumb will be to set a timer—debug actively for forty five–60 minutes, then take a five–ten minute split. Use that point to move all over, stretch, or do a thing unrelated to code. It may come to feel counterintuitive, Particularly underneath tight deadlines, nonetheless it actually contributes to a lot quicker and simpler debugging in the long run.
In a nutshell, having breaks just isn't an indication of weak spot—it’s a smart tactic. It gives your brain Room to breathe, increases your perspective, and will help you steer clear of the tunnel vision That usually blocks your development. Debugging is actually a psychological puzzle, and relaxation is part of solving it.
Understand From Each individual Bug
Each bug you come across is a lot more than simply a temporary setback—It really is a chance to mature as a developer. Regardless of whether it’s a syntax mistake, a logic flaw, or a deep architectural concern, each can train you a little something valuable in the event you make time to mirror and assess what went Completely wrong.
Start by asking your self several essential inquiries when the bug is solved: What brought on it? Why did it go unnoticed? Could it have already been caught earlier with far better procedures like unit testing, code evaluations, or logging? The answers frequently reveal blind spots in your workflow or comprehending and assist you to Develop more powerful coding routines moving forward.
Documenting bugs may also be a superb behavior. Maintain a developer journal or maintain a log in which you Take note down bugs you’ve encountered, the way you solved them, and Whatever you realized. With time, you’ll start to see styles—recurring difficulties or prevalent problems—which you can proactively steer clear of.
In team environments, sharing Anything you've learned from website the bug using your peers can be Primarily highly effective. Regardless of whether it’s through a Slack information, a short write-up, or A fast information-sharing session, assisting Many others stay away from the exact same difficulty boosts staff efficiency and cultivates a stronger Mastering tradition.
More importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll start appreciating them as necessary elements of your enhancement journey. All things considered, some of the finest developers are certainly not the ones who publish perfect code, but individuals who continuously master from their blunders.
Eventually, Each and every bug you deal with adds a whole new layer to your ability established. So next time you squash a bug, take a minute to replicate—you’ll come away a smarter, extra capable developer on account of it.
Summary
Enhancing your debugging capabilities usually takes time, follow, and tolerance — however the payoff is big. It would make you a more effective, self-confident, and able developer. Another time you're knee-deep within a mysterious bug, recall: debugging isn’t a chore — it’s a possibility to become far better at That which you do.